Add images in ListView (C#)
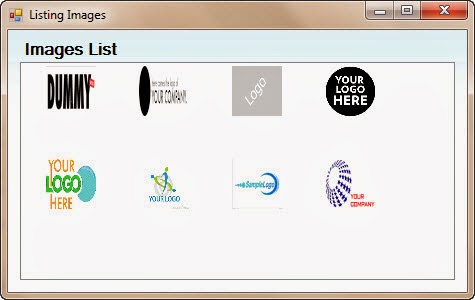
We'll develop an application in which we'll get all images from a directory and add those images in a ListView. Open visual studio and create new " Windows Form Application " project. Go to Toolbox and drag and drop a ListView. Change name of that ListView to " imageListView ". Double at form and it'll navigate you to its load event. Now add following code in form load event. private void Form1_Load(object sender, EventArgs e) { ImageList imageList = new ImageList(); // Clear imageList and imageListView this.imageListView.Items.Clear(); // Get directory info DirectoryInfo dir = new DirectoryInfo(@"D:\Images"); // Read all files in directory foreach (FileInfo file in dir.GetFiles()) { try ...